This is another excerpt from this summer’s 31-page intern project report (PDF). All of this was written entirely by our interns, with only some editing feedback from professional Oasis Digital developers or managers.
Backbone.js
Backbone.js is a JavaScript framework that is loosely based on the model-view-controller (MVC) design. Backbone.js utilizes a RESTful interface when interacting with the server. It is lightweight and is designed for developing single-page applications [16]. There are three main entities used in Backbone: models, collections, and views. Models are objects with attributes that generally represent the data on the server. Collections are arrays of models with added functionality such as filtering and re-ordering. Views represent the UI and hold data containing what should be displayed in the DOM. Views are given either plain HTML or Underscore.js templates. The templates are then filled with data for the model associated with the specific view. It is possible and very common to nest views. Functions can be bound to models or collections to interact with a view when a certain event is called. An example of this in Cerberus is re-rendering the TaskView when the task collection is reset. Backbone.js provides a client-side routing system which uses a hashtag in the URL when navigating pages, opposed to redirecting to a new URL. The DOM is replaced with new elements generated from templates in a view. The DOM is replaced with each route function which gives a fluid look when navigating pages. This is efficient because the developer can update in the DOM he or she wants and does not have to waste resources reloading preexisting content. Backbone.js is a very useful tool for creating simple single-page applications. For applications with a lot of dynamic data it is probably not the best solution. It leaves much to be wanted in terms of model-view interactions. The developer is forced to do more work than should be necessary to tie all of the aspects of the application together. Once everything is tied together, Backbone works exceptionally well. In the future we might explore Angular as an alternative.
gRaphael
gRaphael.js is an extension to a JavaScript drawing library called Raphael. gRaphael.js has limited documentation which makes it hard to use in the beginning. After looking at the available examples, we tweaked some base examples which taught us how to use the extension. Since gRaphael is an extension of Raphael, a Raphael object must first be made [17]. A Raphael object is an HTML element which uses SVG drawings on a canvas to create a surface on the screen that the rest of the plugin can render within. There are four different types of graphs supported by gRaphael: line, pie, bar, and dot. To use one of the types of graphs the corresponding JavaScript file has to be imported in addition to Raphael and gRaphael. To use a graph, we called the graph’s method on the newly created Raphael object and specified the location within the canvas, size, and data to use. There are also specific hover functions that work out of the box with gRapheal [18]. All of the graphs look relatively nice at default settings, but if the end goal is to have different axis labels or highly customized graphs, this library is not the answer.
Example graphs from demos:
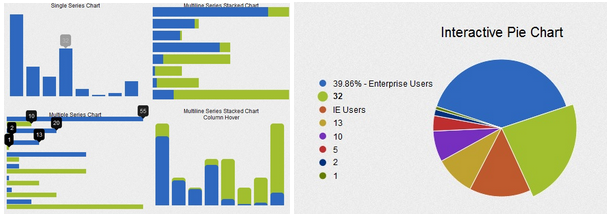
jQuery Mobile Datebox
jQuery Mobile Datebox is a plugin for jQuery Mobile that provide simple ways for users to interact with dates and times. They provide nine different options for date and time selectors. For our project, we used CalBox and TimeBox on the add and edit task pages [19].
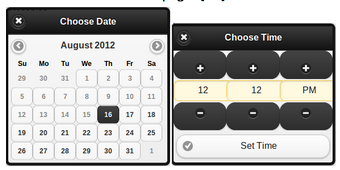
JQM Datebox was very easy to implement into our project. The plugin comes with numerous options that can be set to meet your exact needs. For example, we configured the date-picker to not allow users to select dates in the past. For our project, we envisioned having just one combined input for the date and time. This was surprisingly easy to implement using this plugin because it accepts callback functions for different events. We gave the plugin a callback function for when each dialog is closed. The callback for the date-picker will open the time-picker; the callback for the time-picker will save the user-input into the Backbone model and update the database. It is very easy to format the dates that the user selects. For our project, we formatted the date and time into ISO format for easy compatibility with the database.
jQuery UI Touch Punch
jQuery UI Touch Punch is a JavaScript library that allows jQuery UI widgets to work with touch events on mobile devices. It works by simulating a mouse event when the touch event equivalent is triggered. The library only needs to be included in the head of the HTML page and it will automatically intercept touch events and trigger the correct mouse events. jQuery UI will then use those mouse events just as it would in a normal desktop environment [20]. The core functionality of dragging task divs would not be possible on mobile devices without jQuery UI Touch Punch.
Moment.js
Moment.js is a JavaScript library which provides the capability to format and parse dates. Any JavaScript Date or JSON data can be made into a “moment.” Moment.js allows manipulation, parsing, formatting, or validation of the date. In our project, the date on each task div is formatted by Moment.js to display as text similar to “in twelve days” or “three hours ago,” in order to show when a task is due [21]. Moment.js is also used on the Task Edit page to display a more comprehensive format of the due dates show on the task divs. The Moment.js dates are used in conjunction with unformatted dates that are saved and retrieved from the PostgreSQL database.
Zepto.js
Zepto.js is a JavaScript library that attempts to provide a majority of the functionality of jQuery in a much smaller package. The minified production version of JQuery is 92 KB, and the development version is a much larger 256 KB. Compare this to Zepto.js, which boasts a 24 KB minified size and a mere 48 KB for the full version [22]. One of the goals of the project was to create an application that loads quickly in any browser. With this in mind, we tried to implement Zepto.js first. However, as the project grew, we decided to use the jQuery Mobile plugin, which requires jQuery. Zepto.js is very good for smaller projects or projects that do not require all of the features or plugins of jQuery.
Underscore.js
“Underscore is a utility-belt library for JavaScript that provides a lot of the functional programming support that you would expect in Prototype.js (or Ruby), but without extending any of the built-in JavaScript objects. It’s the tie to go along with jQuery’s tux, and Backbone.js’s suspenders” [23]. Underscore.js contains many functions that simplify the usage of collections and arrays such as forEach, map, filter, every, and indexOf. Underscore is backwards compatible with browsers; it will fall back to native implementations of its functions when requirements are not present in the browser. Underscore comes prepackaged with Backbone.js [23]. We used Underscore’s templating capabilities in our Jade files to display a model attribute directly from the Backbone.js model. The team wrote templates for each Backbone view which were loaded using Underscore’s template function. It would require a fair amount of work to get Underscore to update every time a model was changed, so we settled on re-rendering views when model or collections attributes are changed. Underscore is also used in our filtering/sorting of tasks on both the home and manage pages.
Python
Python is a general purpose scripting language with a very simple rational syntax, allowing for the quick creation of readable programs. Much of its usability is derived from its indentation based syntax, interpreted run time environment, and ability to support numerous programming styles. Despite it being generally considered a scripting language, it supports a vast array of libraries and can easily be packaged into a standalone application [24]. For our use case, Python was exclusively used to create a large number of randomly generated tasks to be inserted into the database. This helped extensively in testing the robustness and reliability of the application.
BCrypt
BCrypt is a Node.js module that can be used to hash passwords for security. BCrypt appends a salt to the password which is a randomly generated string of characters. We used this module to protect all user passwords in the database. BCrypt is a hashing functional, meaning passwords are unable to be decrypted [25]. When we need to check if a user-input matches the password in the database, on the login page for example, we hash the user-inputted text and compare the hash to what is stored in the database. The Node.js BCrypt module requires a C++ compiler, which made it unavailable when we tried to host the project on Windows Azure. BCrypt worked very well other than the prerequisites needed for compiling.
Express.js
Express.js is a Node.js module that creates a web application framework which creates more simple and less redundant server code. There are three main aspects of Express: setting up the application, requesting information, and responding to requests. When setting up the application, the developer can set a port and configure the view engine and routing preferences. The team set the view engine to use Jade, rather than HTML, for our project. The requests to the server determine what page is shown or if a query to the database needs to occur. Requests contain information about the requester such as what they are requesting, data sent with the request, and the URL the request was sent to. The server can then respond with the correct information, sending or displaying information in the form of a response [26].
Jade.js
Jade is a templating engine heavily influenced by Haml. Jade is a different way of writing HTML. It makes creating HTML content easier to write and read. Like Python, Jade uses indentation to denote nested data, instead of curly braces like in JavaScript. For our project, Jade drastically reduced the amount of code we needed to write for our HTML. It provides inline JavaScript which helps to create dynamic web pages. Jade is implemented via a Node.js module. This means that all of the client-side HMTL is compiled on the server and is then sent back to the client. Jade also supports mixins and includes, functions that output HTML and imports of other .jade files, respectively. This does not always have to be the case because Jade supports client-side compiling [27].
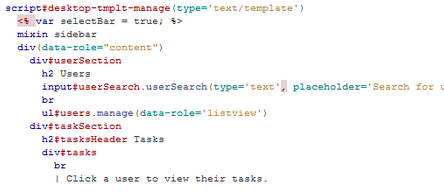
CSS
CSS is the third major pillar to web-development, along with HTML and JavaScript. CSS is used for the styling of the website. CSS is an acronym for Cascading Style Sheets. HTML elements are styled based on their classes or id. Some of the more difficult styling with this project came from the use of jQuery Mobile, a JavaScript library that adds classes to HTML elements before the page is rendered. This hindered our ability to change the styling of certain elements for our own needs. The main parts of our custom css are used to make the task divs colorful with rounded edges and to override part of jQuery Mobile in order to get a look closer to what we wanted.
jQuery Password Strength Meter
jQuery Password Strength Meter is a jQuery plugin that provides realtime client-side evaluation of a password, providing a visual indication regarding the strength of the password. The plugin can be used for any password input field and can be configured to have different tiers of security. It displays a simple, yet informative, progress bar showing the relative security of your entered password.
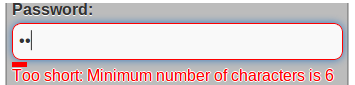
For this project we used the plugin for all of our password input fields. This plugin provides no real addition to the functionality of our application, it simply helps to encourage users to choose stronger passwords.
jQuery Mobile SimpleDialog2
jQuery Mobile provides excellent support for displaying information as a modal dialog. This is a pop-up dialog box that the user must interact with before returning to the main page content. JQM utilizes a routing type system for this, creating the new modal dialog as a “separate page” with a hashtag in the URL, which means the user does not actually leave the page. In our project, this was a major inconvenience. We had to disable jQuery Mobile’s routing system because we chose to use Backbone’s routing system instead. This meant that the modal dialogs would not work with our setup. After many hours of research, we finally found SimpleDialog2, a jQuery Mobile plugin. It provides all of the same functionality of a normal JQM dialog, except it doesn’t use a hashtag in the URL. In fact, it doesn’t need to change the URL at all! Surprisingly enough, it worked on all devices we tested it on. The developer is able to choose one of three options for configuration: button list mode, button input mode, and freeform mode. Button list mode is a simple way of listing input buttons, button input mode is a way of having text input fields, and freeform mode is a way to insert your own HTML into the dialog [28]. We used freeform mode to create a jQuery Mobile listview of users whenever user selection is needed.
The list items are generated from options of a hidden select menu which is generated from the Backbone collection of users.
(to be continued)